If you’re diving into the world of Salesforce development, one of the first things you’ll need to master is querying data using SOQL (Salesforce Object Query Language) and SOSL (Salesforce Object Search Language). These two languages are at the heart of Salesforce’s data retrieval processes, and understanding how to use them efficiently is crucial for optimizing your application’s performance. Today, I’ll walk you through the essentials of SOQL and SOSL, share some handy query optimization tips, and point out common pitfalls to avoid. By the end of this post, you’ll be better equipped to write effective and efficient queries in your Salesforce projects.
What is SOQL in Salesforce Development?
SOQL is Salesforce’s own version of SQL, designed specifically for querying Salesforce data. If you’re familiar with SQL, you’ll find SOQL relatively easy to pick up. The key difference is that SOQL is used exclusively to query data from Salesforce objects, whereas SQL can query data from multiple tables in relational databases.
In Salesforce development, SOQL is your go-to tool for retrieving records from Salesforce objects like Accounts, Contacts, Opportunities, and Custom Objects. A basic SOQL query might look something like this:
sql
Copy code
SELECT Name, Phone FROM Account WHERE Industry = ‘Technology’
This query retrieves the Name and Phone fields from all Account records where the Industry field is set to ‘Technology.’ Simple enough, right? But as your application grows and the volume of data increases, you’ll need to think more critically about how your queries are structured to maintain performance.
What is SOSL & When Should You Use It?
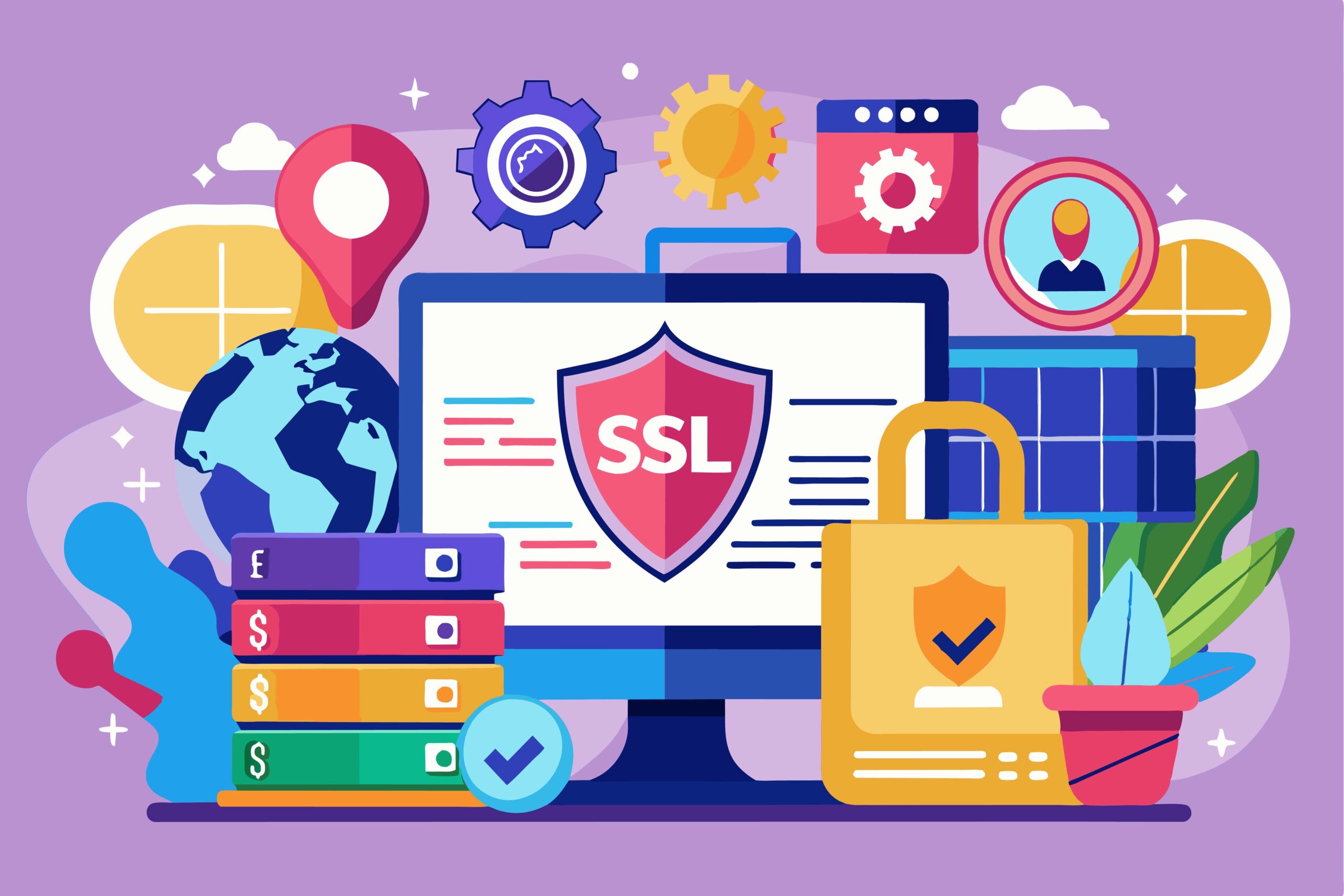
While SOQL is great for pinpointing specific records, SOSL shines when you need to perform a broader search across multiple objects. Think of SOSL as a search engine for your Salesforce org. It allows you to search across text fields, such as Name, Phone, and Email, across multiple objects, all with a single query.
Here’s an example of a basic SOSL query:
sql
Copy code
FIND ‘Acme*’ IN Name Fields RETURNING Account(Name), Contact(FirstName, LastName)
This query searches for records where the Name field contains “Acme” and returns matching Account and Contact records. SOSL is especially useful when you need to search across different objects simultaneously or when you’re looking for a specific term in multiple fields.
Optimization Tip
1. Use Selective SOQL Queries
In Salesforce development, SOQL query optimization starts with making your queries selective. A selective query is one that retrieves only the records you need, minimizing the data load on your org. To make your SOQL queries more selective, use indexed fields in your WHERE clause. Indexed fields, like the record ID or custom fields marked as external IDs, can significantly speed up your queries by reducing the number of records Salesforce needs to scan.
For example, instead of querying all records and filtering them in your application, you can filter them directly in your SOQL query:
sql
Copy code
SELECT Id, Name FROM Account WHERE CreatedDate = LAST_N_DAYS:30 AND Industry = ‘Technology’
This query is more efficient because it uses indexed fields to filter records before returning them, reducing the amount of data processed.
2. Bulkify Your Queries
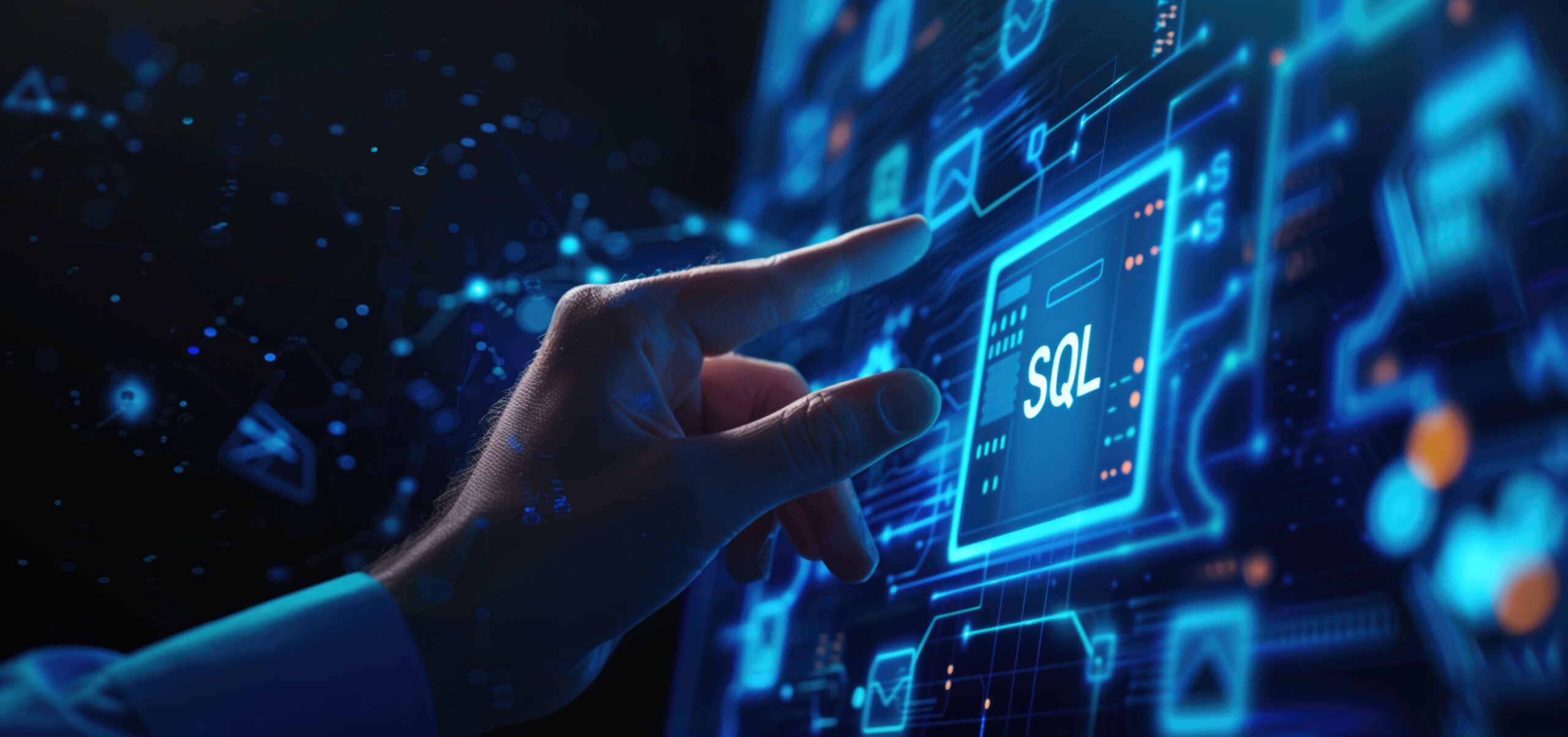
When you’re working in Salesforce development, one of the biggest mistakes you can make is running SOQL queries inside a loop. This can lead to hitting governor limits, which are the limits Salesforce imposes to ensure fair use of shared resources.
Instead of querying the database multiple times in a loop, bulkify your queries to handle multiple records at once. Here’s an example of how you can do this:
sql
Copy code
List<Account> accounts = [SELECT Id, Name FROM Account WHERE Id IN :accountIds];
By bulkifying your query, you retrieve all necessary records in a single transaction, which is more efficient and reduces the likelihood of hitting governor limits.
3. Know When to Use SOSL Over SOQL
In Salesforce development, it’s essential to know when to use SOQL and when to use SOSL. While SOQL is perfect for retrieving specific records based on exact field values, SOSL should be your go-to for keyword searches across multiple objects or fields.
If your use case involves searching for a term across multiple objects or fields, SOSL is more efficient. For example, if a user enters a keyword in a search box and you need to find matching records across Accounts, Contacts, and Opportunities, SOSL can perform this search in one go, while SOQL would require multiple queries.
However, be cautious with SOSL. While it’s powerful, it can also be more resource-intensive than SOQL. Only use SOSL when you truly need to search across multiple objects or fields. If you’re querying a specific field within a single object, SOQL is likely the better choice.
4. Leverage Query Plan Tool
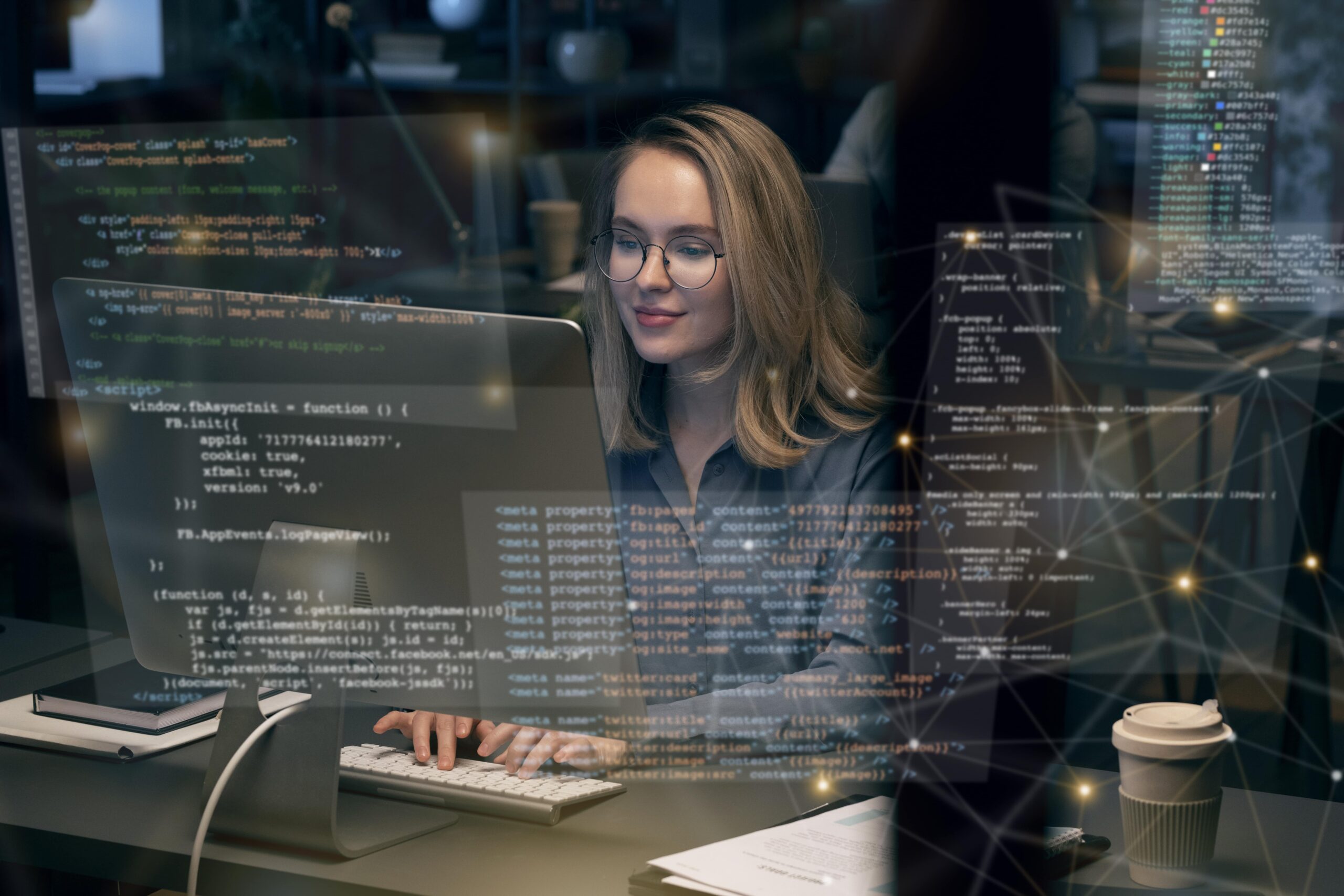
Salesforce provides a Query Plan Tool that helps you understand how your SOQL queries are being processed. This tool is a must-use for any Salesforce developer looking to optimize their queries. It gives you insight into the cost of your query and whether Salesforce is using any indexes to optimize it.
The Query Plan Tool displays a cost number for each execution path, where a lower cost is better. By reviewing the query plan, you can make informed decisions on how to tweak your query for better performance. For example, if your query has a high cost, you might consider adding a filter on an indexed field or reducing the number of fields being queried.
Pitfalls in Salesforce Development
1. Ignoring Governor Limits
One of the biggest pitfalls in Salesforce development is ignoring Salesforce’s governor limits. These limits are there to ensure that no single process consumes excessive resources. For SOQL, some key limits include the number of records returned, the number of queries that can be executed, and the total number of DML operations.
Hitting these limits can cause your code to fail, resulting in errors or incomplete processes. To avoid this, always be mindful of governor limits when writing SOQL or SOSL queries. You can check for these limits using the Limits class in Apex, which provides methods to get information about the current limits in your execution context.
2. Not Testing Queries with Real Data
Another common pitfall is not testing your SOQL and SOSL queries with real data. It’s easy to write a query that works well on a small set of test data, only to have it fail or perform poorly when run on a larger data set in production.
To avoid this, always test your queries with data that closely matches the size and structure of your production environment. This will give you a more accurate picture of how your queries will perform when they go live. You can also use Salesforce’s Developer Console or a Sandbox environment to run and optimize your queries before deploying them to production.
Read Customizing Salesforce with Apex: Best Practices & Common Pitfalls for more information.
Conclusion
Understanding and optimizing SOQL and SOSL queries is a crucial skill in Salesforce development. By making your queries selective, bulkifying them, knowing when to use SOSL, and leveraging tools like the Query Plan Tool, you can significantly improve the performance of your Salesforce applications. At the same time, being aware of common pitfalls like ignoring governor limits and not testing with real data can help you avoid costly mistakes.
Remember, efficient queries not only improve performance but also ensure that your Salesforce org remains scalable and responsive as your data grows. With these tips in hand, you’ll be well on your way to mastering SOQL and SOSL, and taking your Salesforce development skills to the next level.
For more insights on technology and innovation, visit TheBrandWick.